Introduction
Hi,This is Asif Khan.In the world of WordPress, plugins are essential for enhancing the functionality of your site. Whether you’re a seasoned developer or a beginner, creating your own plugins can be a powerful way to add unique features to your WordPress site. In this comprehensive guide, we’ll walk you through the process of creating a WordPress plugin that displays the five most recent blog posts. We’ll cover everything from setting up the plugin to styling the output, ensuring you have all the tools you need to implement this feature on your site.
Why Create a Custom Plugin for Recent Blog Posts?
Before diving into the technical details, let’s explore why creating a custom plugin for displaying recent blog posts can be beneficial:
- Tailored Functionality: Custom plugins allow you to add specific features that meet your exact needs. Unlike pre-built plugins, you can control every aspect of your plugin’s functionality and design.
- Performance Optimization: By creating a plugin tailored to your site’s requirements, you can ensure it is optimized for performance, reducing the load on your site and improving user experience.
- Learning Opportunity: Developing your own plugin is an excellent way to enhance your WordPress development skills and gain a deeper understanding of how WordPress works.
Step 1: Setting Up Your Plugin
1. Create the Plugin Folder and File
To start, you need to create a folder and a PHP file for your plugin:
- Navigate to
wp-content/plugins
in your WordPress installation directory. - Create a new folder named
recent-blog-posts
. - Inside this folder, create a file named
recent-blog-posts.php
.
2. Add Plugin Header Information
Open recent-blog-posts.php
and add the following code. This header tells WordPress about your plugin:
<?php
/*
Plugin Name: Recent Blog Posts
Description: Displays the latest 5 blog posts using a shortcode.
Version: 1.0
Author: Asif Khan
*/
This header contains essential information such as the plugin name, description, version, and author.
Step 2: Register the Shortcode
Shortcodes in WordPress allow you to insert dynamic content into posts and pages. Here’s how to create a shortcode for displaying recent blog posts.
1. Define the Shortcode Function
Add the following function to recent-blog-posts.php
. This function queries the latest blog posts and formats them for display:
function rbs_recent_blog_posts($atts) {
// Default attributes
$atts = shortcode_atts(array(
'number' => 5, // Number of posts to display
), $atts, 'recent_blog_posts');
// Query arguments
$args = array(
'post_type' => 'post',
'posts_per_page' => intval($atts['number']),
'order' => 'DESC',
'orderby' => 'date',
);
// The Query
$query = new WP_Query($args);
// Check if there are posts
if ($query->have_posts()) {
$output = '<ul class="recent-blog-posts">';
while ($query->have_posts()) {
$query->the_post();
$output .= '<li><a href="' . get_permalink() . '">' . get_the_title() . '</a></li>';
}
$output .= '</ul>';
// Restore original Post Data
wp_reset_postdata();
return $output;
} else {
return '<p>No posts found.</p>';
}
}
Explanation:
shortcode_atts()
: Sets default attributes for the shortcode. In this case, the default number of posts is set to 5.WP_Query
: Retrieves the most recent blog posts according to the specified arguments.have_posts()
,the_post()
: Loops through the posts.get_permalink()
,get_the_title()
: Fetches the post link and title.
2. Register the Shortcode
To make your shortcode available for use in WordPress, add this code:
add_shortcode('recent_blogs_posts', 'rbs_recent_blog_posts');
This registers the [recent_blogs_posts]
shortcode, which you can use in any post or page to display the recent blog posts.
Step 3: Enqueue External CSS
Styling your plugin output enhances its appearance and integration with your site’s design.
1. Create and Style Your CSS
Inside your plugin folder, create a file named style.css
. Add the following CSS code to style the list of recent blog posts:
/* General styles for the recent blog posts list */
.recent-blog-posts {
list-style-type: none;
padding: 0;
margin: 0;
border-radius: 16px;
box-shadow: 0 12px 24px rgba(0, 0, 0, 0.3);
background: linear-gradient(135deg, #0f2027, #2c5364);
overflow: hidden;
font-family: 'Roboto', sans-serif;
color: #fff;
}
/* Styles for individual blog post items */
.recent-blog-posts li {
padding: 20px;
border-bottom: 1px solid rgba(255, 255, 255, 0.2);
transition: background-color 0.3s, transform 0.3s, box-shadow 0.3s, border-color 0.3s, transform 0.3s;
border-radius: 12px;
position: relative;
overflow: hidden;
background: rgba(0, 0, 0, 0.3); /* Semi-transparent background */
}
/* Remove the border from the last item */
.recent-blog-posts li:last-child {
border-bottom: none;
}
/* Add a futuristic background effect to each item */
.recent-blog-posts li::before {
content: '';
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: linear-gradient(135deg, rgba(0, 255, 255, 0.1), rgba(255, 0, 255, 0.1));
transition: opacity 0.3s;
z-index: 0;
}
/* Styles for links */
.recent-blog-posts a {
text-decoration: none;
color: #00bcd4; /* Neon blue */
font-size: 22px;
font-weight: 700;
display: block;
position: relative;
transition: color 0.3s, transform 0.3s, letter-spacing 0.3s, text-shadow 0.3s;
letter-spacing: 1px;
z-index: 1;
}
/* Hover effects for list items and links */
.recent-blog-posts li:hover {
background-color: rgba(255, 255, 255, 0.1);
transform: translateY(-8px) scale(1.03);
box-shadow: 0 20px 40px rgba(0, 255, 255, 0.4);
border-color: rgba(255, 255, 255, 0.3);
}
.recent-blog-posts li:hover::before {
opacity: 0;
}
.recent-blog-posts a:hover {
color: #ff4081; /* Neon pink */
text-decoration: underline;
letter-spacing: 1.2px;
text-shadow: 0 4px 8px rgba(0, 0, 0, 0.3);
transform: scale(1.05);
}
/* Add a sleek animation for the hover effect */
.recent-blog-posts li {
animation: fadeIn 0.8s ease-in-out, scaleUp 0.8s ease-in-out;
}
/* Keyframes for fade-in and scale-up animations */
@keyframes fadeIn {
from {
opacity: 0;
transform: translateY(20px);
}
to {
opacity: 1;
transform: translateY(0);
}
}
@keyframes scaleUp {
from {
transform: scale(0.98);
}
to {
transform: scale(1);
}
}
/* Responsive adjustments */
@media (max-width: 768px) {
.recent-blog-posts {
border-radius: 12px;
box-shadow: 0 10px 20px rgba(0, 0, 0, 0.25);
}
.recent-blog-posts a {
font-size: 20px;
}
}
@media (max-width: 480px) {
.recent-blog-posts {
border-radius: 8px;
padding: 10px;
}
.recent-blog-posts li {
padding: 15px;
}
.recent-blog-posts a {
font-size: 18px;
}
}
Explanation:
.recent-blog-posts
: Styles the overall list, including border-radius, box-shadow, and background gradient..recent-blog-posts li
: Styles individual list items with padding, borders, and transitions.::before
: Adds a gradient overlay effect for a modern look.a
: Styles the links with color, size, and hover effects.
2. Enqueue the CSS File
To include this CSS file in your plugin, add the following code to recent-blog-posts.php
:
function rbs_enqueue_styles() {
wp_enqueue_style('recent-blog-posts-style', plugins_url('style.css', __FILE__));
}
add_action('wp_enqueue_scripts', 'rbs_enqueue_styles');
This code ensures that your CSS file is loaded on the front end of your site.
Step 4: Testing Your Plugin
1. Activate the Plugin
Go to the WordPress admin dashboard, navigate to Plugins, and activate your “Recent Blog Posts” plugin.
2. Use the Shortcode
Add the [recent_blogs_posts]
shortcode to any post or page where you want to display the most recent blog posts.
3. Check the Front End
Visit the page where you’ve added the shortcode and verify that the list of recent blog posts appears correctly.
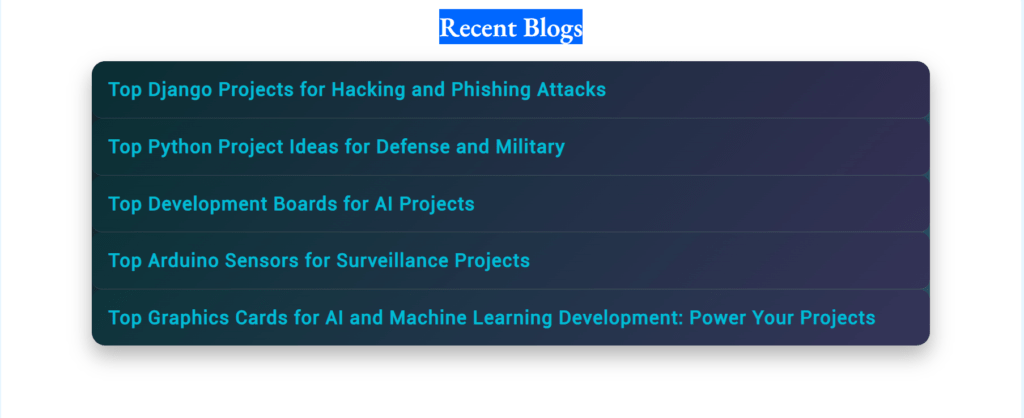
Step 5: Troubleshooting and Enhancements
1. Common Issues
- No Posts Displayed: Ensure that you have published blog posts on your site. If there are no posts, the plugin will display “No posts found.”
- Styling Issues: Double-check that your
style.css
file is correctly enqueued and that the CSS rules are applied.
2. Enhancements
- Customizable Number of Posts: Modify the shortcode to accept a custom number of posts.
- Advanced Styling: Experiment with additional CSS properties to match your site’s theme.
Conclusion
Creating a WordPress plugin to display the five most recent blog posts is a rewarding project that can enhance your site’s functionality and design. By following this guide, you’ve learned how to set up a basic plugin, register a shortcode, and style the output with custom CSS.
Feel free to customize and extend your plugin further to meet your specific needs. With these skills, you’re well on your way to becoming a more proficient WordPress developer.
For more tutorials and insights on WordPress and other web development topics, check out my blog, subscribe to my YouTube channel, and follow me on Instagram for updates!